The strategy method performs different functionality depending on its provided internals.
i.e. Car car = new Car(new HybridEngine(), new BrakeDisc());
This way, the same object class can be used, and reused with different behaviour
Code
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
| interface Engine {
void vroom();
void performMaintenance();
}
class DieselEngine implements Engine {
void vroom() { System.out.println("gdrgdgrdgrgdgrgdgrg"); };
void performMaintenance() { ... };
}
class ElectricEngine implements Engine {
void vroom() { System.out.println("-------------------"); };
void performMaintenance() { ... };
}
class Car {
private Engine engine;
public MyCar(Engine e) {
this.engine = e;
}
void vroom() {
this.engine.vroom();
}
void performMaintenance() {
this.engine.performMaintenance();
}
}
///
Car car1 = new Car(new DieselEngine()); // gdrgdgrdgrgdgrgdgrg
Car car2 = new Car(new ElectricEngine()); // -------------------
|
UML
Interfaces are joined to their contexts through a solid line with a white diamond on the context side.
Implementations of the interface are joined through a dotted line with a white triangle on the interface.
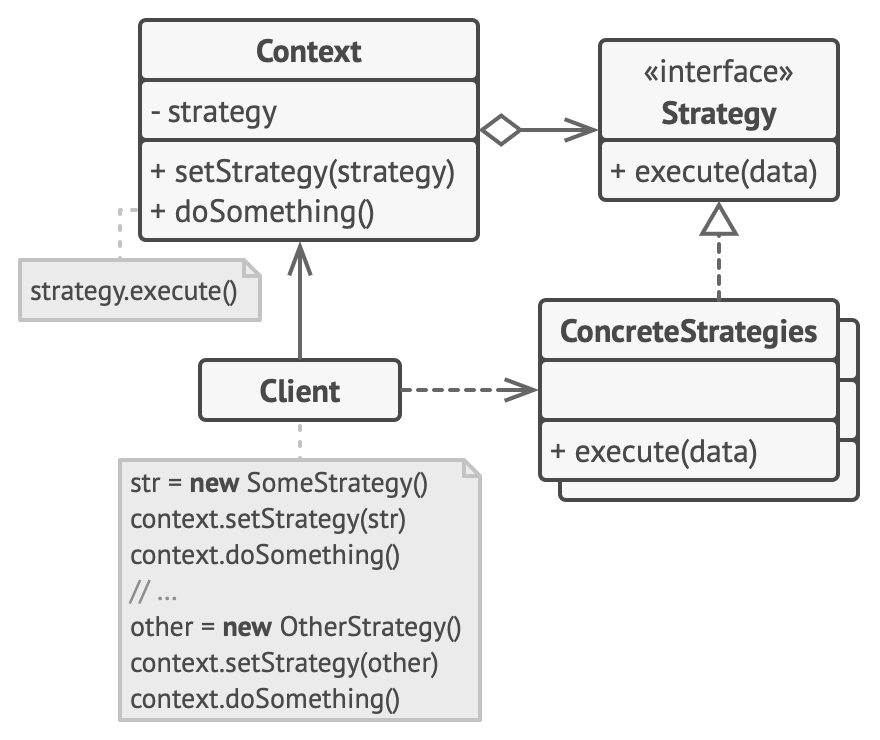